Hướng dẫn tạo dịch vụ lập lịch trình chuyến đi bằng GPT-3 và Streamlit
Tác giả: Bếp Hạ Thảo
Thời gian phát hành: 2024-12-30 17:55:39
Số lượt xem: 1051
🚀 Yêu cầu cần thiết
Đầu tiên, chúng ta cần cài đặt các thư viện cần thiết:
!pip install openai
!pip install streamlit
!pip install python-dotenv
Bây giờ, chúng ta có thể tạo một tệp .env
và thêm mã API OpenAI của mình:
OPENAI_API_KEY=<your api key>
Cuối cùng, chúng ta cần tạo tệp main.py
, nhập tất cả các thư viện cần thiết và tải mã API từ tệp .env
:
import os
import random
from datetime import datetime, timedelta
import openai
import streamlit as st
from dotenv import load_dotenv
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
📝 Tạo ứng dụng Streamlit
Bây giờ, chúng ta có thể tạo ứng dụng Streamlit. Đầu tiên, chúng ta cần tạo một hàm để tạo prompt và lịch trình chuyến đi dựa trên đầu vào của người dùng:
example_destinations = ['Paris', 'London', 'New York', 'Tokyo', 'Sydney', 'Hong Kong', 'Singapore', 'Warsaw', 'Mexico City', 'Palermo']
random_destination = random.choice(example_destinations)
now_date = datetime.now()
# làm tròn đến 15 phút gần nhất
now_date = now_date.replace(minute=now_date.minute // 15 * 15, second=0, microsecond=0)
# tách thành đối tượng ngày và giờ
now_time = now_date.time()
now_date = now_date.date() + timedelta(days=1)
def generate_prompt(destination, arrival_to, arrival_date, arrival_time, departure_from, departure_date, departure_time, additional_information, **kwargs):
return f'''\nPrepare trip schedule for {destination}, based on the following information:\n\n* Arrival To: {arrival_to}\n* Arrival Date: {arrival_date}\n* Arrival Time: {arrival_time}\n\n* Departure From: {departure_from}\n* Departure Date: {departure_date}\n* Departure Time: {departure_time}\n\n* Additional Notes: {additional_information}\n'''.strip()
def submit():
prompt = generate_prompt(**st.session_state)
# tạo đầu ra
output = openai.Completion.create(
engine='text-davinci-003',
prompt=prompt,
temperature=0.45,
top_p=1,
frequency_penalty=2,
presence_penalty=0,
max_tokens=1024
)
st.session_state['output'] = output['choices'][0]['text']
Bây giờ chúng ta có thể tạo giao diện người dùng cho ứng dụng bằng Streamlit:
# Khởi tạo
if 'output' not in st.session_state:
st.session_state['output'] = '--'
st.title('GPT-3 Trip Scheduler')
st.subheader('Let us plan your trip!')
Hãy tạo một biểu mẫu cho đầu vào của người dùng:
with st.form(key='trip_form'):
c1, c2, c3 = st.columns(3)
with c1:
st.subheader('Destination')
origin = st.text_input('Destination', value=random_destination, key='destination')
st.form_submit_button('Submit', on_click=submit)
with c2:
st.subheader('Arrival')
st.selectbox('Arrival To', ('Airport', 'Train Station', 'Bus Station', 'Ferry Terminal', 'Port', 'Other'), key='arrival_to')
st.date_input('Arrival Date', value=now_date, key='arrival_date')
st.time_input('Arrival Time', value=now_time, key='arrival_time')
with c3:
st.subheader('Departure')
st.selectbox('Departure From', ('Airport', 'Train Station', 'Bus Station', 'Ferry Terminal', 'Port', 'Other'), key='departure_from')
st.date_input('Departure Date', value=now_date + timedelta(days=1), key='departure_date')
st.time_input('Departure Time', value=now_time, key='departure_time')
st.text_area('Additional Information', height=200, value='I want to visit as many places as possible! (respect time)', key='additional_information')
Cuối cùng, chúng ta có thể hiển thị lịch trình chuyến đi được tạo ra:
st.subheader('Trip Schedule')
st.write(st.session_state.output)
🚀 Chạy ứng dụng Streamlit
Bây giờ chúng ta có thể chạy ứng dụng:
!streamlit run main.py
🏆 Kết quả
Hãy kiểm tra kết quả!
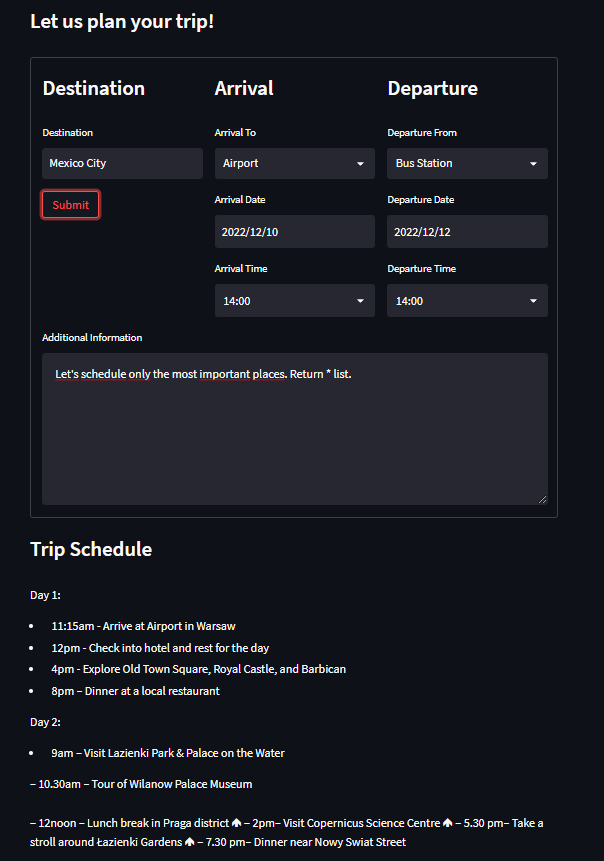
Link gốc: lablab.ai